Cloud Chaos to Cloud Control: Locking Down Terraform Deployments
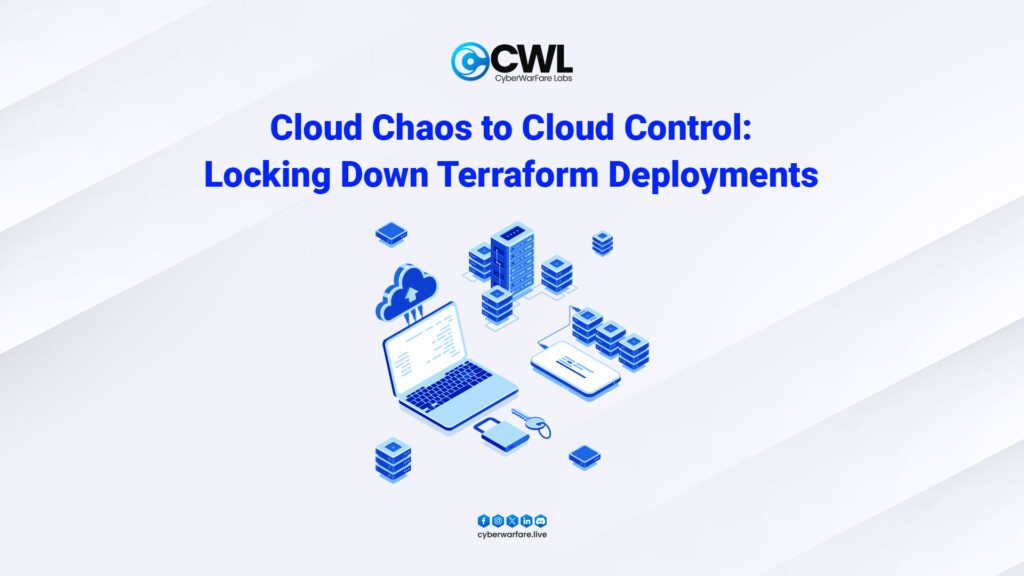
Terraform has emerged as the de facto standard for Infrastructure as Code (IaC), enabling cloud engineers and DevOps professionals to automate infrastructure deployment efficiently. However, improper Terraform configurations can introduce significant security risks, including data exposure, privilege escalation, and misconfigured cloud resources.
In this blog, we will explore Terraform, its common security pitfalls, best practices, and hands-on demonstrations across AWS, Azure, and GCP to help you secure your cloud infrastructure effectively.
Terraform allows you to define, provision, and manage cloud and on-prem resources in a declarative manner. Below are some key Terraform concepts:
Providers
- Providers are plugins that interact with cloud services (AWS, Azure, GCP) or other APIs.
- Example:
provider "aws" {
region = "us-east-1"
}
Variables
- Used to make Terraform configurations dynamic and reusable.
- Example:
variable "region" {
default = "us-east-1"
}
Resources
- Resources define infrastructure components like EC2 instances, S3 buckets, IAM roles, etc.
- Example:
resource "aws_instance" "example" {
ami = "ami-12345678"
instance_type = "t2.micro"
}
Data Sources
- Fetches external data that can be used within Terraform.
- Example:
data "aws_ami" "latest_ami" {
most_recent = true
owners = ["amazon"]
}
Output Values
- Used to extract and display information after execution.
- Example:
output "instance_ip" {
value = aws_instance.example.public_ip
}
State Files (terraform.tfstate)
- Stores the current state of deployed infrastructure.
- Helps Terraform track changes and perform updates.
Terraform Commands
- terraform init → Initializes the working directory.
- terraform plan → Shows what changes Terraform will apply.
- terraform apply → Provisions the infrastructure.
- terraform destroy → Destroys all managed resources.
Common Terraform Security Pitfalls
1. Hardcoded Secrets in Terraform Code (Azure Example)
Risk: Directly storing sensitive configurations, credentials, or API keys in Terraform scripts leaves them vulnerable to source code repository leaks.
Example (Risky):
provider "azurerm" {
features {}
subscription_id = "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
client_id = "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
client_secret = "SuperSecretClientSecret1234"
tenant_id = "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"
}
Solution: Use Azure Key Vault to store secrets securely and reference them in Terraform.
provider "azurerm" {
features {}
subscription_id = var.azure_subscription_id
client_id = var.azure_client_id
client_secret = data.azurerm_key_vault_secret.client_secret.value
tenant_id = var.azure_tenant_id
}
data "azurerm_key_vault_secret" "client_secret" {
name = "terraform-client-secret"
key_vault_id = "/subscriptions/xxxx/resourceGroups/xxxx/providers/Microsoft.KeyVault/vaults/xxxx"
}
2. Overly Permissive IAM Roles and Policies (AWS Example)
Risk: Attackers may be able to access cloud resources without authorization if IAM roles and policies are assigned in an excessively permissive manner.
Example of a risky IAM policy:
resource "aws_iam_policy" "admin_policy" {
name = "admin-policy"
policy = jsonencode({
Version = "2012-10-17",
Statement = [{
Effect = "Allow",
Action = "*",
Resource = "*"
}]
})
}
Solution: Follow the Principle of Least Privilege by granting only the required permissions.
resource "aws_iam_policy" "limited_policy" {
name = "limited-policy"
policy = jsonencode({
Version = "2012-10-17",
Statement = [{
Effect = "Allow",
Action = ["s3:ListBucket", "s3:GetObject"],
Resource = ["arn:aws:s3:::my-secure-bucket", "arn:aws:s3:::my-secure-bucket/*"]
}]
})
}
3. Exposing Sensitive Outputs (GCP Example)
Risk: Exposure of logs or Terraform state files may result in security breaches when sensitive data is displayed in Terraform outputs.
Example (Risky):
output "database_password" {
value = google_sql_database_instance.default.settings[0].password
}
Solution: Mark sensitive outputs to prevent them from being displayed.
output "database_password" {
value = google_sql_database_instance.default.settings[0].password
sensitive = true
}
Terraform Security Best Practices
1. Enable State Encryption and Remote Storage (AWS Example)
Terraform state files often contain sensitive/private information. Keeping them locally may put your security at risk.
Best practice: Terraform state should be remotely stored in a safe location, like AWS S3, with DynamoDB enabled for state locking.
terraform {
backend "s3" {
bucket = "my-secure-tf-state"
key = "terraform.tfstate"
region = "us-east-1"
encrypt = true
dynamodb_table = "terraform-lock"
}
}
2. Implement Policy-as-Code with Terraform Sentinel or OPA (Azure Example)
Security policies can be enforced across Terraform configurations by using policy-as-code tools like Open Policy Agent (OPA) or HashiCorp Sentinel.
Example OPA policy to restrict Azure Storage Containers from being public:
package terraform.azure_storage
deny[msg] {
input.resource_type == "azurerm_storage_container"
input.resource.public_access == "blob"
msg = "Azure Storage Containers must not have public access enabled."
}
3. Use Automated Security Scanning Tools (GCP Example)
Several tools can help identify misconfigurations in Terraform scripts:
- Checkov: Static code analysis for Terraform security best practices.
- tfsec: Scans Terraform code for security misconfigurations.
- Terrascan: Enforces security best practices on Terraform code.
Example of running tfsec on a GCP Terraform script:
To download tfsec (Bash script – Linux):
curl -s https://raw.githubusercontent.com/aquasecurity/tfsec/master/scripts/install_linux.sh | bash
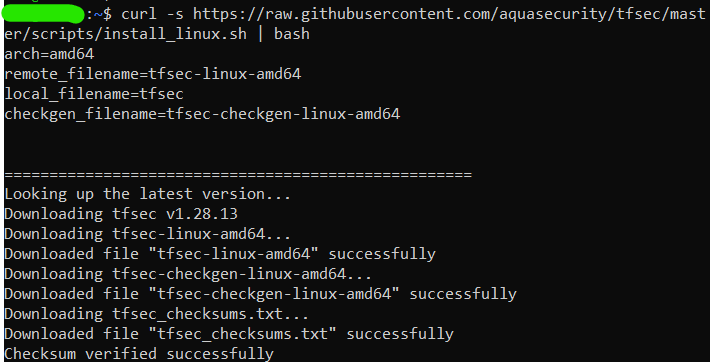
tfsec .
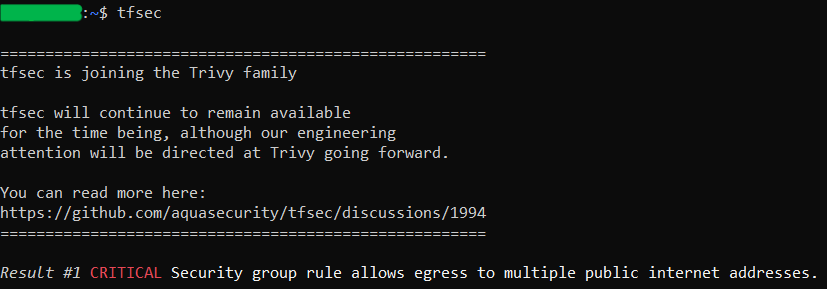
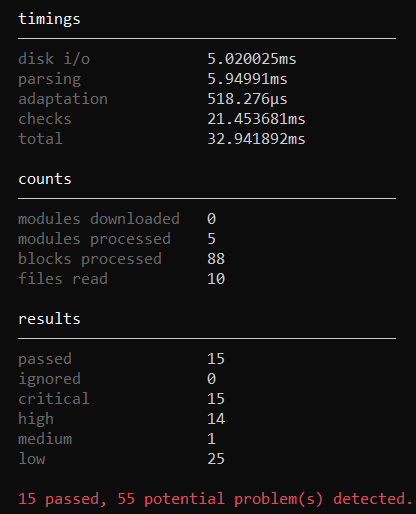
4. Limit Resource Exposure with Network Security Groups (AWS Example)
Ensure that Terraform-created cloud resources follow the least-exposure principle.
Example of a misconfigured security group:
resource "aws_security_group" "insecure_sg" {
ingress {
from_port = 22
to_port = 22
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
}
Secure version:
resource "aws_security_group" "secure_sg" {
ingress {
from_port = 22
to_port = 22
protocol = "tcp"
cidr_blocks = ["192.168.1.0/24"]
}
}
Conclusion
You can greatly strengthen the security posture of your Terraform-managed cloud infrastructure by putting these best practices into effect. Avoid hardcoding secrets, implement security principles, adhere to the least privilege principle, and routinely check Terraform setups for vulnerabilities.
Although Terraform is an effective tool, there are significant hazards to cloud security if proper security precautions are not taken. Be proactive, think about security, and use automated tools to test your Terraform scripts on a regular basis.
Want to learn more? Keep an eye on the CybeWarFare Labs Blog Section [Here] for further insights into cloud security best practices!